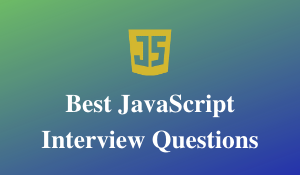
What are the advantages of using JavaScript?
Lightweight: JavaScript can be executed within the user’s browser without having to communicate with the server, saving on bandwidth.
Versatile: JavaScript supports multiple programming paradigms—object-oriented, imperative, and functional programming and can be used on both front-end and server-side technologies.
Sleek Interactivity: Because tasks can be completed within the browser without communicating with the server, JavaScript can create a smooth “desktop-like” experience for the end user.
Rich Interfaces: From drag-and-drop blocks to stylized sliders, there are numerous ways that JavaScript can be used to enhance a website’s UI/UX.
Prototypal Inheritance: Objects can inherit from other objects, which makes JavaScript so simple, powerful, and great for dynamic applications.
Explain the difference between classical inheritance and prototypal inheritance?
The great thing about JavaScript is the ability to do away with the rigid rules of classical inheritance and let objects inherit properties from other objects.
Classical Inheritance: A constructor function instantiates an instance via the “new” keyword. This new instance inherits properties from a parent class.
Prototypal Inheritance: An instance is created by cloning an existing object that serves as a prototype. This instance—often instantiated using a factory function or “Object.create()”—can benefit from selective inheritance from many different objects.
Give an example of a time that you used functional programming in JavaScript?
Functional programming is one of the key paradigms that makes JavaScript stand out from other languages. Look for examples of functional purity, first-class functions, higher-order functions, or using functions as arguments and values. It’s also a good sign if they have past experience working with functional languages like Lisp, Haskell, Erlang, or Clojure.
Give an example of a time that you used Prototypal OO in JavaScript?
Prototypal OO is the other major programming paradigm that really lets JavaScript shine—objects linked to other objects (OLOO). You’re looking for knowledge of when and where to use prototypes, liberal use of “Object.assign()” or mixins, and a solid grasp of concepts like delegation and concatenative inheritance.
What is a RESTful Web Service?
REST stands for Representational State Transfer, an architectural style that has largely been adopted as a best practice for building web and mobile applications. RESTful services are designed to be lightweight, easy to maintain, and scaleable. They are typically based on the HTTP protocol, make explicit use of HTTP methods (GET, POST, PUT, DELETE), are stateless, use intuitive URIs, and transfer XML/JSON data between the server and the client.
Which frameworks are you most familiar with?
You can tell a lot about a programmer from the frameworks they’re familiar with—AngularJS, React, jQuery, Backbone, Aurelia, and Meteor are just some of the more popular ones available. The key here is to make sure the developer you’re engaging has experience with the framework you’ve chosen for your project.
How experienced are you with MEAN?
The MEAN (MongoDB, Express, AngularJS, and Node.js) stack is the most popular open-source JavaScript software stack available for building dynamic web apps—the primary advantage being that you can write both the server-side and client-side halves of the web project entirely in JavaScript. Even if you aren’t intending to use MEAN for your project, you can still learn a lot about the developer when they recount their experiences using JavaScript for different aspects of web development.
difference between document.ready and anonymous function in javascript
Anonymous function will be executed firs.
Self-invoking functions runs instantly i.e will be executed as soon as it is encountered in the Javascript. $(document).ready(function() {})(); will trigger only after dom elements are completely constructed.
How to you change the title of the page by javascript?
document.title = “new title”;
Are you a team player? Give an example of a time when you had to resolve a conflict with another member on your team.
There are many jobs associated with putting together an application, and chances are high that your new JavaScript developer will at the very least have to interface with a designer. You’re looking for a developer who can communicate effectively when they need to, responds to emails, and knows how to coordinate with other branches of a project.
Determine the output of the code below. Explain your answer.
var lorem = { ipsum : 1};
var output = (function(){
delete lorem.ipsum;
return lorem.ipsum;
})();
console.log(output);
The output would be undefined, because the delete operator removed the property “ipsum” from the object “lorem” before the object was returned. When you reference a deleted property, the result is undefined.
Explain the differences between one-way data flow and two-way data binding?
This question may seem self-explanatory, but what you’re looking for is a developer who can demonstrate solid understanding of how data flows throughout the application. In two-way data binding, changes to the UI and changes to the model occur asynchronously—a change on one end is reflected on the other. In one-way data binding, data only flows one way, and any changes that the user makes to the view will not be reflected in the model until the two are synced. Angular makes implementing two-way binding a snap, whereas React would be your framework of choice for deterministic one-way data flow.
What is the output of 10+20+”30″ in JavaScript?
3030 because 10+20 will be 30. If there is numeric value before and after +, it is treated is binary + (arithmetic operator).
Difference between Client side JavaScript and Server side JavaScript?
Client side JavaScript comprises the basic language and predefined objects which are relevant to running java script in a browser. The client side JavaScript is embedded directly by in the HTML pages. This script is interpreted by the browser at run time. Server side JavaScript also resembles like client side java script. It has relevant java script which is to run in a server. The server side JavaScript are deployed only after compilation.
In which location cookies are stored on the hard disk?
The storage of cookies on the hard disk depends on OS and the browser.
The Netscape navigator on Windows uses cookies.txt file that contains all the cookies. The path is : c:\Program Files\Netscape\Users\username\cookies.txt
The Internet Explorer stores the cookies on a file username@website.txt. The path is: c:\Windows\Cookies\username@Website.txt.