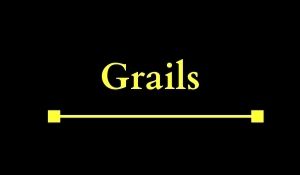
1.What are the best practices to be followed while developing a grails application?
Building an application using the grails framework is quite easy. But, when building it the right way we need to follow the best practices. Some of the practices followed while building a grails application are listed below:
•Controller: the controller logic should be as simple as possible. Duplication of code should be avoided.
•Service: it is the right choice for a coarse-grained code. By default, services are transactional in nature.
•Views: views should be as simple as possible. A consistent look should be maintained throughout the pages of the application.
•Internationalization: all the text messages in view should be moved to “messages.properties”.
•Domain: the specific logic of the model domain should be placed in its own domain.
2.What do you mean by Scaffolding? How do we use it?
Scaffolding is used to generate some basic CRUD interfaces for a domain class. It includes the necessary views and some controller actions for creating, updating, reading, and deleting CRUD operations. There are basically two types of scaffolding-
•Static scaffolding: with the help of this, controllers and views can be generated to create certain interfaces using the command line.
•Dynamic scaffolding: using dynamic scaffolding, various functions like- index, show, edit, etc. can be dynamically implemented by default by using the runtime scaffolding mechanism.
For expressing a dependency on the scaffolding plugin, we can use-“build.gradle”.
3.What is the use of bootstrap.groovy?
Sometimes a situation occurs in which a particular task is to be carried out every time the application starts or stops.”bootstrap.groovy” is used for this particular situation. It is a simple plain groovy script which has two important closures: the “init” and “destroy” files. So, whenever the application starts or stops, these closures are called upon respectively. This “bootstrap.groovy” is present inside the grails-app/conf folder. It is used to define a code whenever the application performs any function.
4.What do you mean by closures?
A closure is a short and anonymous block of code which is just used to span a few lines of code. This block of code can even be entered as a parameter in the method. Closures are anonymous in nature. Formal parameters can also be contained in closures so that it can be more useful just like methods in groovy. Closures can also refer to a variable at the time when a closure is defined. Closures can be a type of parameter for a method. Few methods provided by closure itself are listed below:
•find()
•findAll()
•any() and every()
•Collect (), etc.
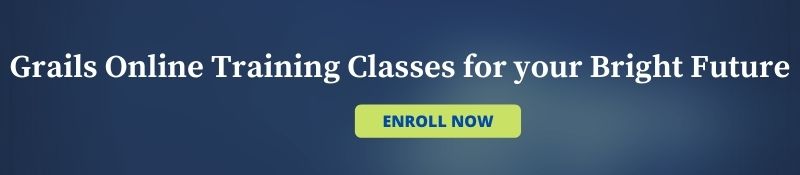
5.What do you understand by metaprogramming?
Metaprogramming is the ability of adding new methods or variables to the classes dynamically at run time. You can add methods or variables whenever and wherever you want. This is a very powerful ability. Be it the use of code production, unit tests, or anything in between, these capabilities increases the curiosity of the java developers. These are the programs that write or manipulate other programs. These are responsible for dynamic string execution. These help in exposing the internal runtime engine to the programming codes through API’s. The meta object protocols make the program semantics explicit and extensible.
6. I have a parameter in my Config.groovy file named layer1.prop1. How can I access this in a controller? How can I access this in a service?
In a controller, I can inject grailsApplication via def grailsApplication and access this variable via grailsApplication.config.layer1.prop. Anywhere else, I can use the ConfigurationHolder and access it via ConfigurationHolder.config.layer1.prop1
7. How do I edit the web.xml file of my grails application?
First, I need to generate the template files via grails install-templates. The web.xml template will be under src/templates/war directory.
Alternatively, I could also create a plugin and use the doWithWebDescriptor method to add or remove nodes from my web.xml file.
8. Explain the concept of externalized configuration. What is this good for?
•Externalized configurations let me define other config and .properties files that don’t live within the grails-app/conf directory.
•To enable this, I need to define locations for the application to look for configuration files via the grails.config.locations in Config.groovy in relation to the classpath or filesystem.
•The most popular uses of externalized configurations is to enable configuration changes without having to rebuild a war file. By planning ahead, configurations can even be reloaded without restarting the application container via this mechanism.
9.Explain the difference between the Config.groovy and BuildConfig.groovy files.
•The Config.groovy file contains configuration properties and information needed when running your application. It contains properties used by your services, controllers, etc.
•The BuildConfig.groovy file contains information relevant to building and deploying your application, such as repositories, jar file dependencies and war file name.
10. What is the difference between the Grails console, shell and interactive modes?
•Grails interactive is interactive mode of the grails command line interface. It lets you run Gant scripts in the script runner one after another.
•Grails console is a Swing-based command console similar to the Groovy console. It let’s you execute code against a full environment that has access to all your domain classes and quickly test out code that would go into controllers and services.
•Grails shell is the headless version of Grails console. This is useful when you want to access and test out code in a remote SSH-based server. One of the advantages of the Grails shell is that it does not reload when domain classes change like the console does, so it is useful also for long-running scripts, although the new run – script command in Grails 1.3.6 might be better suited for those.
11. How do I get a hold of my Spring beans in the Grails console?
Grails console injects a ctx variable, which represents the Spring context. You can then just use ctx.getBean( ‘myServiceName’ ) to get a hold of your beans.
12. What are some things I need to be careful about when working with the Grails console?
The bootstrap files don’t get loaded. So if your database is in dbCreate = ‘create[-drop]’ mode, you might not get the same data that you would expect.The console also reloads on code change the same way that grails run-app does. So the code that you have typed in might get lost.
13. What happens when you call grails bug-report?
Grails generates a dehydrated timestamped minimal zip file that can be attached to JIRA reports. One way to hydrate these into full projects is to call grails upgrade on them.
14. What is the difference between run-app and run-war?
Run-war will compile and build a war file that it runs from the embedded container ( Tomcat unless you’ve changed it ), run-app runs the application from compiled sources in the file system. This means that any change to controllers, views, etc will be reflected immediately by run-app, but not by run-war. It also means that scripts that get run at application packaging time ( such as the ui-performance plugin’s zip and minify process ) won’t happen at run-app time.
15. How do I create a custom script in Grails?
Call grails create-script myPackage.myScript . This will create a new Gant script file under the scripts directory that you can then run via grails my-script.
16. What is the _Events.groovy file? What can it be used for?
This file allows us to define event hooks that get called whenever grails scripts fire events. For example, if I wanted to have a utility method that gets fired whenever compilation is finished, I can add it to the CompileEnd method in _Events.groovy.
17. Explain the different options available to dbCreate.
•Not having a dbCreate / null – don’t mess with the database
•create-drop- nuke the database and create a new one
•create – if the database is there, don’t change it. Otherwise make a new one.
•update – change the database but keep the data if it is there, create a new one if you can’t find anything.
18. What are dynamic finders?
Dynamic finders are methods that are created automatically based on domain class properties by Grails and GORM. They provide a simple way to query domain classes using the power of groovy metaprogramming.
Example: If my domain class Movie has a date called ReleaseDate, I can call Movie.findByReleaseDateGreaterThan( today ) and GORM will automatically resolve this and generate the correct query in the background.
19. Explain what joinTable does within GORM.
The joinTable domain class mapping allows us to customize the table that gets generated for associations. It lets me change the name, primary key and inverse column used in the database to map a list of primitives, enum, one-to-many or many-to-many relationship.
20. Explain what lock() is in GORM and why you would use it.
Sometimes when many people are working on the same set of data, Hibernate will throw a StaleObjectStateException and die.
The lock() method makes sure that nobody else can change your domain class by issuing a pessimistic lock on the database row until you commit your transaction.
21. What is the difference between calling a read() and a get() in GORM?
read() gets the object in read-only mode. get() retrieves the object and allows it to be modified. One consequence of this is that get() might automatically save changes to the database via hibernate dirty checking while read() will not.
22. What does the removeFrom() function do in a domain object?
Whenever you have a one-many relationship between a and b, calling a.removeFrom(b) destroys the back reference between a and b. This is the reverse of the addTo() method.
23. What are named queries? Where do you define them?
Name queries are aliases written using the criteria builder DSL that allow us to re-use commonly used queries. You put them in the domain classes’ namedQueries static property.
24. What is the difference between a dynamic finder, a HQL query and a criteria?
All three are used to query domain objects.
•Dynamic finders are methods of the form findAllByName( “pancho” ) added to domain classes.
•Criterias are a DSL based on Hibernate Criterias and Groovy builders, they look like this: c { like(“name”, “pancho” ) }.
•HQL is a query language that looks like SQL but is fully object-oriented. It looks like this: “select distinct s.name from Student.s”
25. Explain Gorm events. Provide two examples.
Gorm events are methods that you can insert into your domain objects and are executed before and after the domain object is saved into the database. Two examples are beforeInsert and afterDelete
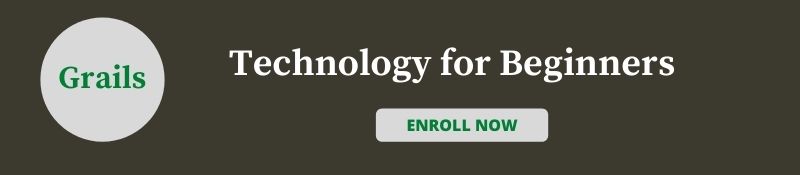
26. What are constraints in Grails? Give me three examples.
Constraints let you define the rules for validating a domain object. They are defined under the static constraints closure in a domain object. Three examples might be nullable, blank and email.
27. Give me two examples of constraints that affect the display within scaffolding views.
•widget: ‘textarea’ will make the field display in a text area instead of a one line input field.
•display: ‘none’ will hide the constraint from being shown.
28. Give me two examples of constraints that affect database generation.
•nullable: true will cause the database schema to mark the column as nullable.
•unique: true will cause the column index to be marked as unique.
•maxSize: number will set the length of the field to the number.
29. What are Grails command objects? How can they be used in validation?
Command objects are intermediate domain objects that are not saved in the database. You should use them whenever you are just interested in updating a subset of properties from a domain object, or when there is a form that does not map one-to-one with a domain object.
Command objects support both validation and data binding, so you can use these to validate the data entered into forms easily.
30. What is the difference between validate() and save()?
Save indicates to hibernate that the object should be persisted, validate simply runs all the validation commands and check it. Save runs validate.